Insider Tips to Master HTTP/2 Server Push
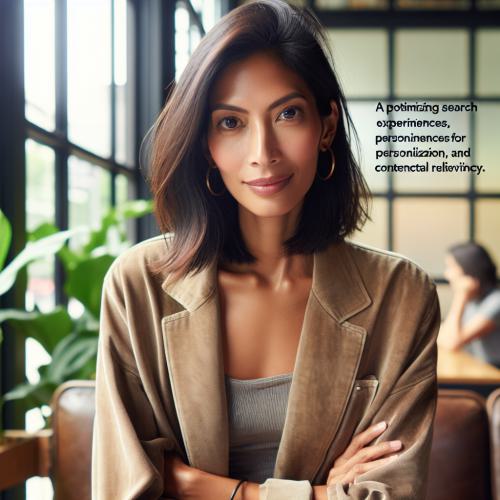
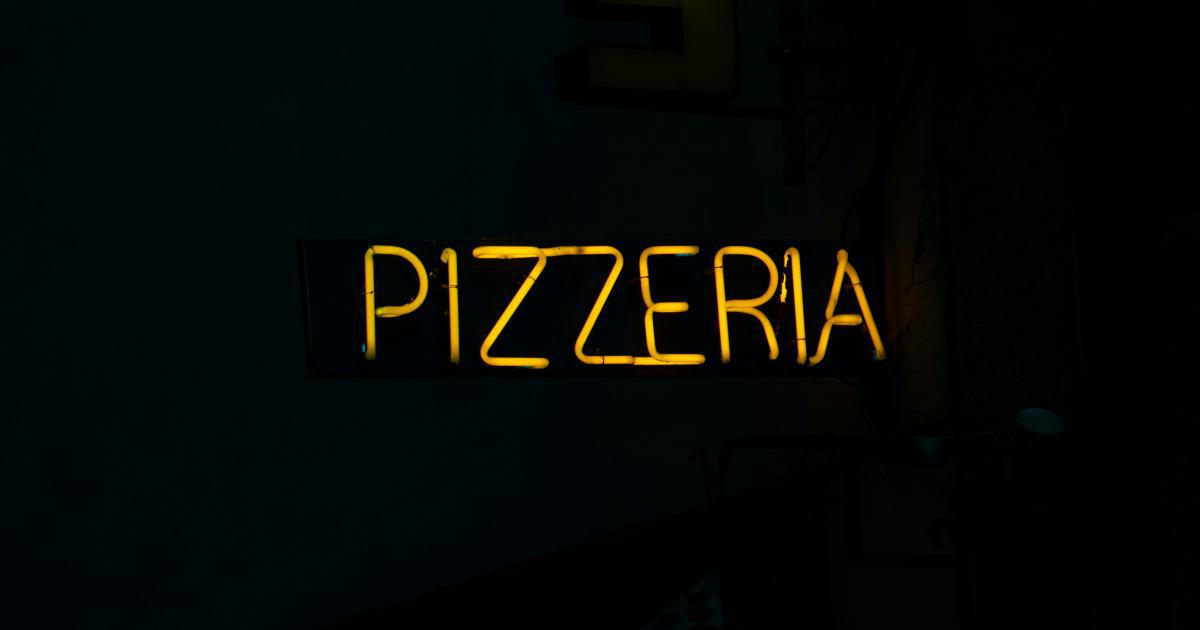
Introduction: Unlocking the Power of HTTP/2 Server Push
In the ever-evolving landscape of web technologies, the recent advancements in HTTP/2 have introduced a game-changing feature known as server push. This innovative capability allows web servers to proactively deliver resources to clients, reducing page load times and improving the overall user experience. As the adoption of HTTP/2 continues to grow, understanding and mastering the intricacies of server push has become a critical skill for web developers and architects.
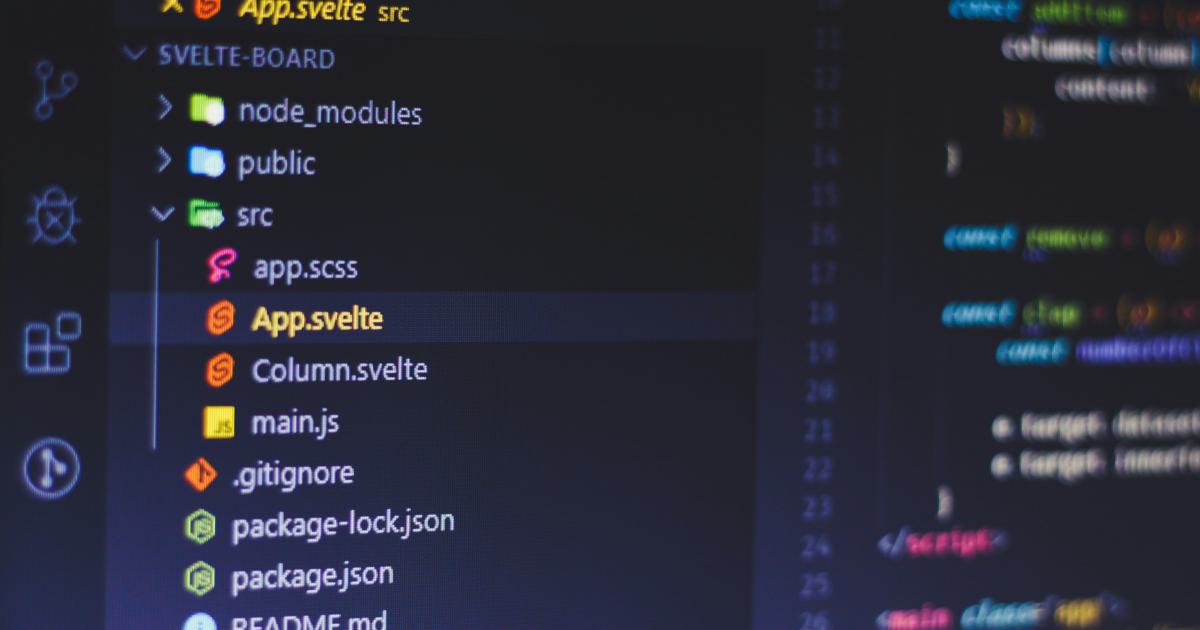
In this comprehensive article, we'll dive deep into the world of HTTP/2 server push, exploring its inner workings, best practices, and practical applications. Whether you're a seasoned web professional or just getting started, this guide will equip you with the knowledge and insights needed to harness the full potential of this powerful technique.
Understanding HTTP/2 Server Push
The Evolution of Web Delivery
The traditional HTTP/1.1 protocol, while a reliable workhorse, has inherent limitations when it comes to modern web application requirements. With the increasing complexity of web pages, the need for faster and more efficient resource delivery has become paramount. This is where the introduction of HTTP/2 has revolutionized the way web content is delivered.
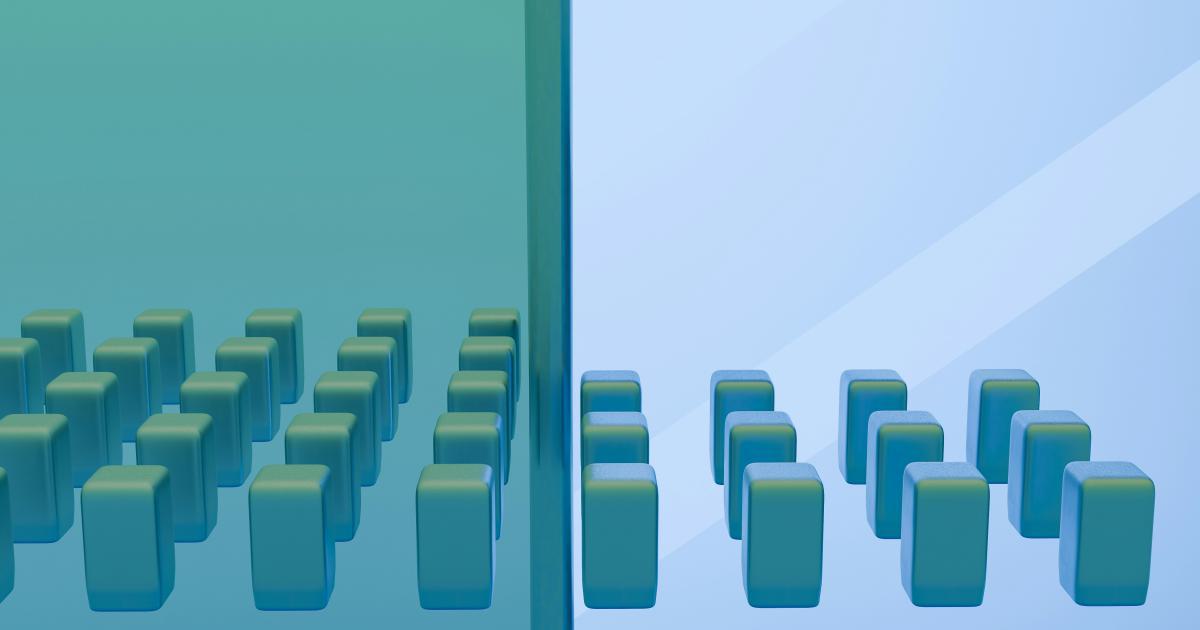
At the core of HTTP/2 is a fundamental shift in the way clients and servers communicate. Instead of the sequential, request-response model of HTTP/1.1, HTTP/2 utilizes a multiplexed approach, allowing multiple resources to be delivered concurrently over a single connection. This architectural change sets the stage for the powerful feature of server push.
What is HTTP/2 Server Push?
HTTP/2 server push is a mechanism that enables web servers to proactively send resources to clients, without waiting for the client to request them. This means that the server can anticipate the client's needs and preemptively deliver resources, such as CSS, JavaScript files, or images, before the client even knows they're needed.
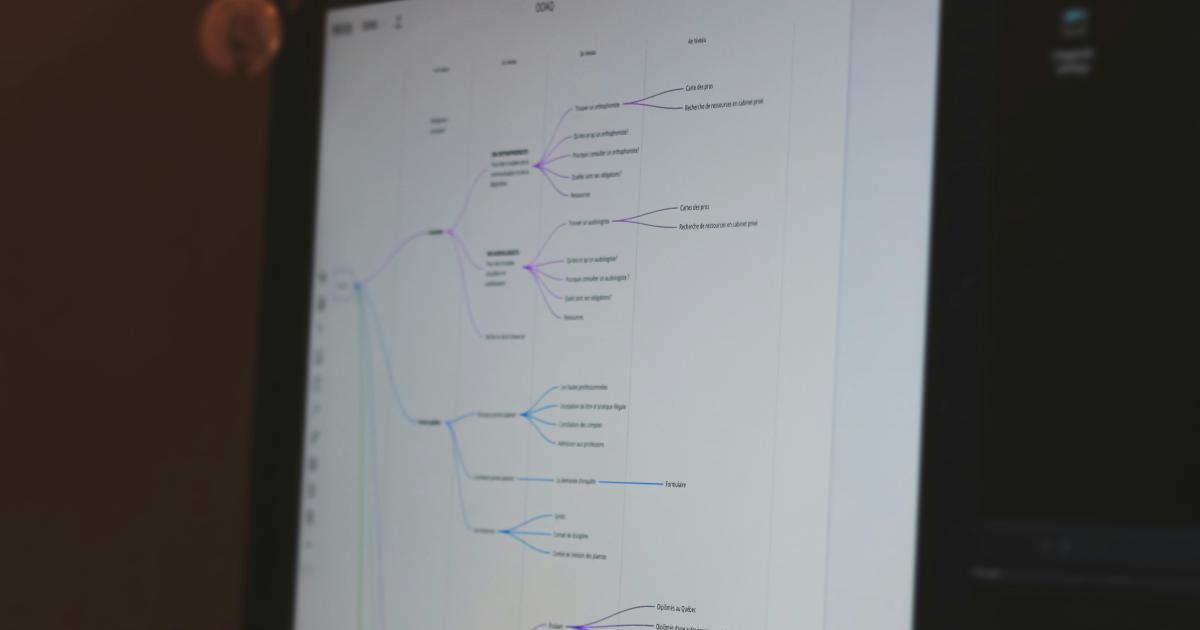
The key benefit of this approach is that it reduces the number of round-trips between the client and server, ultimately leading to faster page load times. By eliminating the need for the client to request each resource individually, server push can significantly improve the perceived performance of a web application.
Understanding Server Push Flow
The server push process typically follows these steps:
Client Requests a Resource: The client initiates a request for a web page or a specific resource.
Server Analyzes the Request: The server examines the client's request and identifies additional resources that the client is likely to need, such as stylesheets, scripts, or images.
Server Pushes the Resources: The server proactively sends these identified resources to the client, without waiting for the client to request them.
Client Receives and Caches the Pushed Resources: The client receives the pushed resources and stores them in its cache for future use.
Client Loads the Requested Resource: The client loads the originally requested resource, utilizing the preemptively delivered resources as needed.
By streamlining this process, server push can significantly reduce the perceived latency experienced by the user, leading to a more responsive and efficient web application.
Implementing HTTP/2 Server Push
Configuring the Server
To enable server push, you'll need to ensure that your web server is configured to support HTTP/2. This typically involves setting up the necessary protocols and certificates on your server. While the specific configuration steps may vary depending on your server software (e.g., Apache, Nginx, or Microsoft IIS), the general process often includes the following:
Enable HTTP/2 Support: Ensure that your server software is configured to support the HTTP/2 protocol.
Obtain Valid SSL/TLS Certificates: Server push requires a secure HTTPS connection, so you'll need to obtain and configure valid SSL/TLS certificates for your web server.
Configure Server Push Settings: Depending on your server software, you'll need to specify the resources that should be proactively pushed to the client. This may involve modifying configuration files or using server-specific directives.
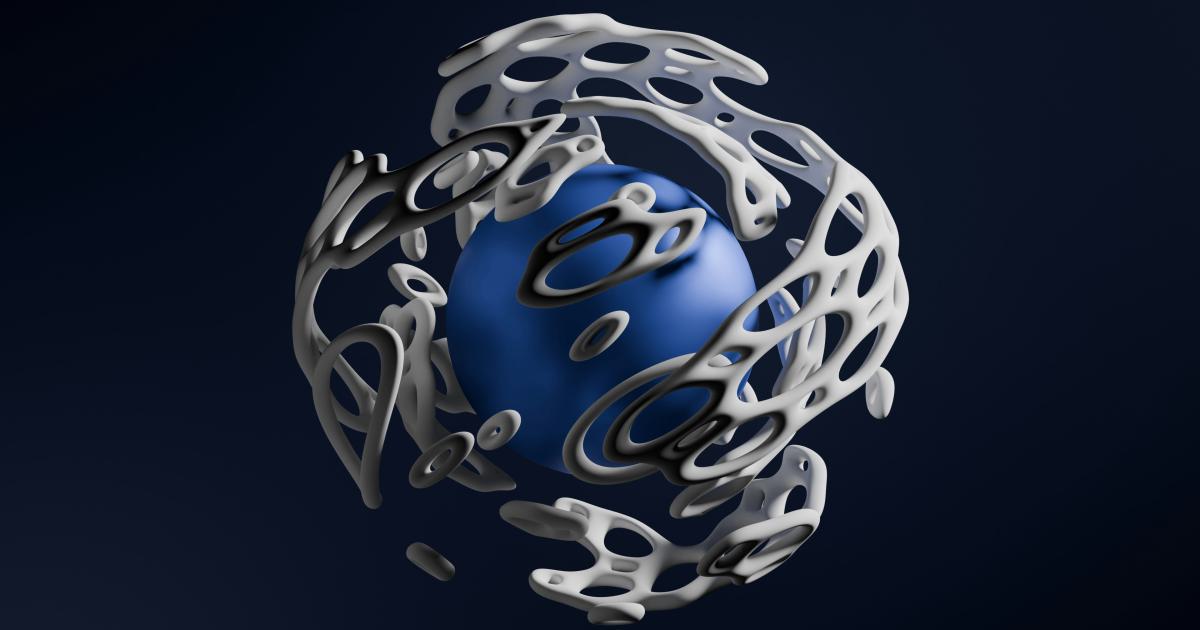
It's important to note that the specific configuration steps may vary depending on your server software and version. Consult the documentation provided by your server vendor for detailed instructions on how to enable and configure HTTP/2 server push.
Identifying Resources to Push
Determining which resources to push is a crucial step in optimizing the server push process. The goal is to identify the resources that are most likely to be needed by the client, without overwhelming the connection with unnecessary data.
Some common strategies for identifying resources to push include:
Analyzing the HTML Response: Examine the HTML response and identify any referenced resources, such as stylesheets, scripts, and images.
Considering the User's Context: Take into account the user's current location within the application and anticipate the resources they are likely to need next.
Leveraging Server-side Intelligence: Utilize server-side analytics or machine learning models to predict the resources that are most commonly requested by users in similar contexts.
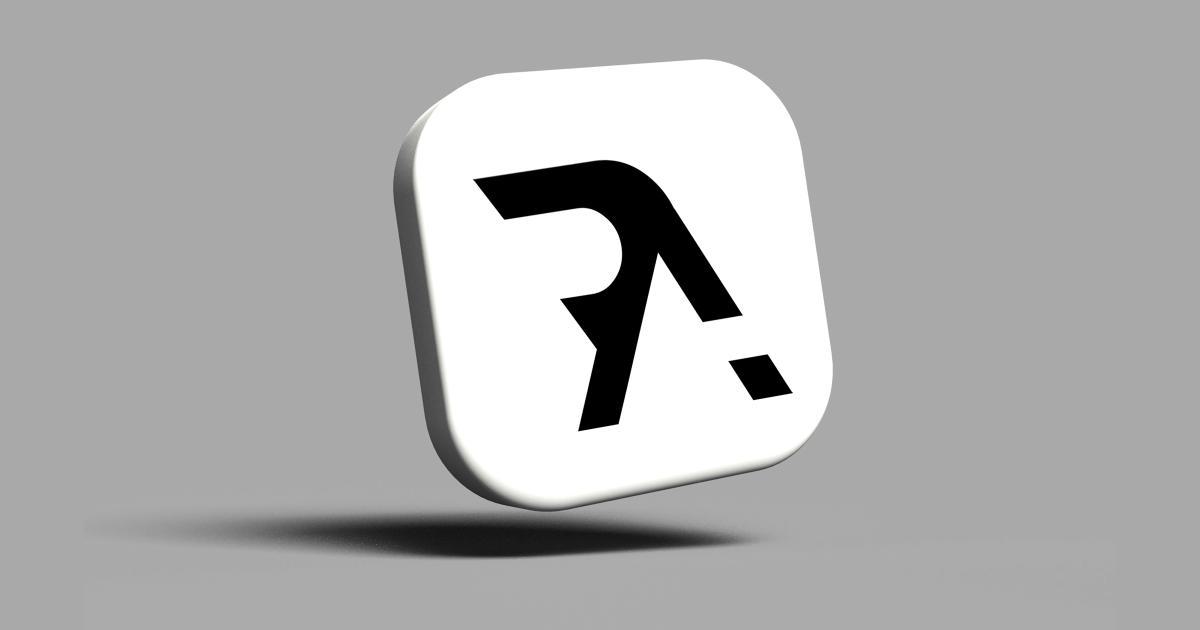
By carefully selecting the resources to push, you can strike a balance between reducing latency and avoiding unnecessary network traffic, leading to a more efficient and responsive web experience.
Implementing Server Push in Code
The specific implementation of server push will depend on the web server software and the programming language or framework you're using. However, the general process typically involves the following steps:
Identify the Resources to Push: As discussed in the previous section, analyze the requested resource and determine which additional resources should be pushed.
Generate the Push Promises: Create "push promises" for each of the resources you want to push. These promises indicate to the client that the server will be delivering the resources proactively.
Send the Push Promises: Before sending the initial response, transmit the push promises to the client.
Push the Resources: Concurrently with the initial response, send the actual pushed resources to the client.
Here's an example of how you might implement server push in a Node.js application using the Express framework:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
// Identify resources to push
const resourcesToPush = ['/styles.css', '/script.js', '/image.jpg'];
// Generate push promises
resourcesToPush.forEach(resource => {
res.push(resource, {
response: {
'content-type': 'text/css'
},
promise: res.push(resource)
});
});
// Send the initial response
res.send('<html><body><h1>Hello, HTTP/2 Server Push!</h1></body></html>');
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
In this example, the server identifies the resources to be pushed, generates the push promises, and sends the initial response along with the pushed resources. The specific implementation details may vary depending on the server software and programming language you're using, but the core concepts remain the same.
Best Practices for HTTP/2 Server Push
As you begin to implement HTTP/2 server push, it's important to follow best practices to ensure optimal performance and efficiency. Here are some key considerations:
Avoid Unnecessary Pushes
While the ability to push resources can be tempting, it's crucial to avoid overdoing it. Pushing too many resources can lead to increased network traffic and potentially even reduce performance if the client is unable to process all the pushed data in a timely manner.
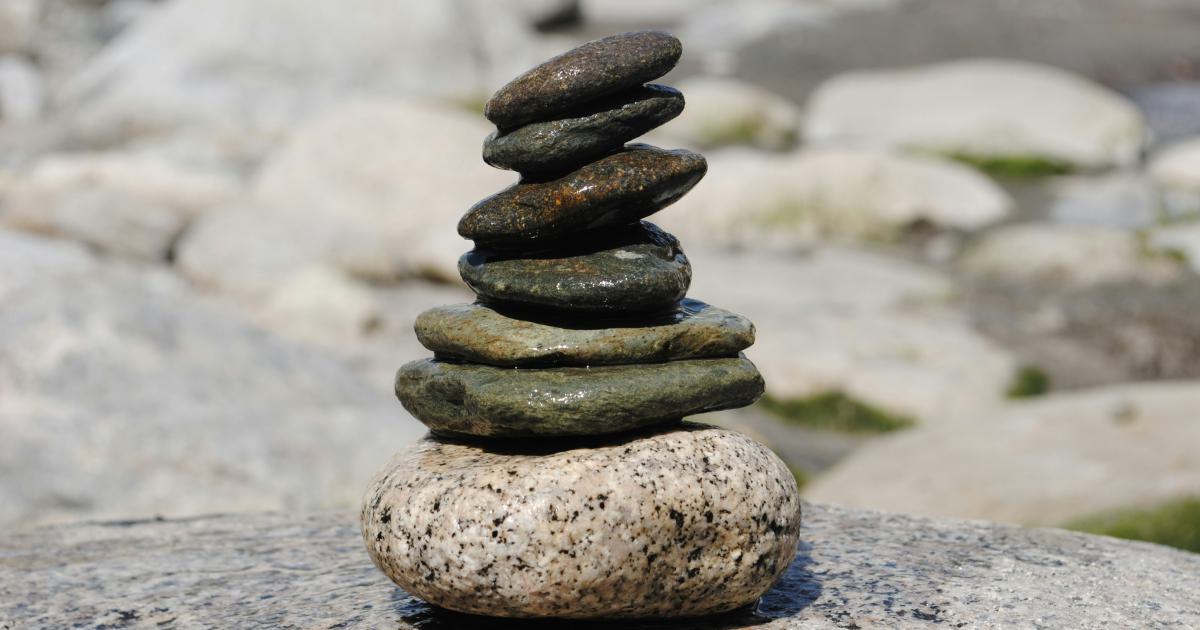
To strike the right balance, carefully analyze the requested resource and only push the most critical assets that the client is likely to need. Regularly review and refine your push strategies to ensure you're not overwhelming the client with unnecessary data.
Leverage Caching and Conditional Requests
Effective caching is a crucial aspect of server push optimization. Ensure that your server is correctly setting appropriate cache-control headers for the pushed resources, allowing the client to reuse them in subsequent requests.
Additionally, consider implementing conditional requests using headers like If-Modified-Since
or If-None-Match
. This allows the client to check if a resource has been updated before downloading it again, reducing unnecessary network traffic.
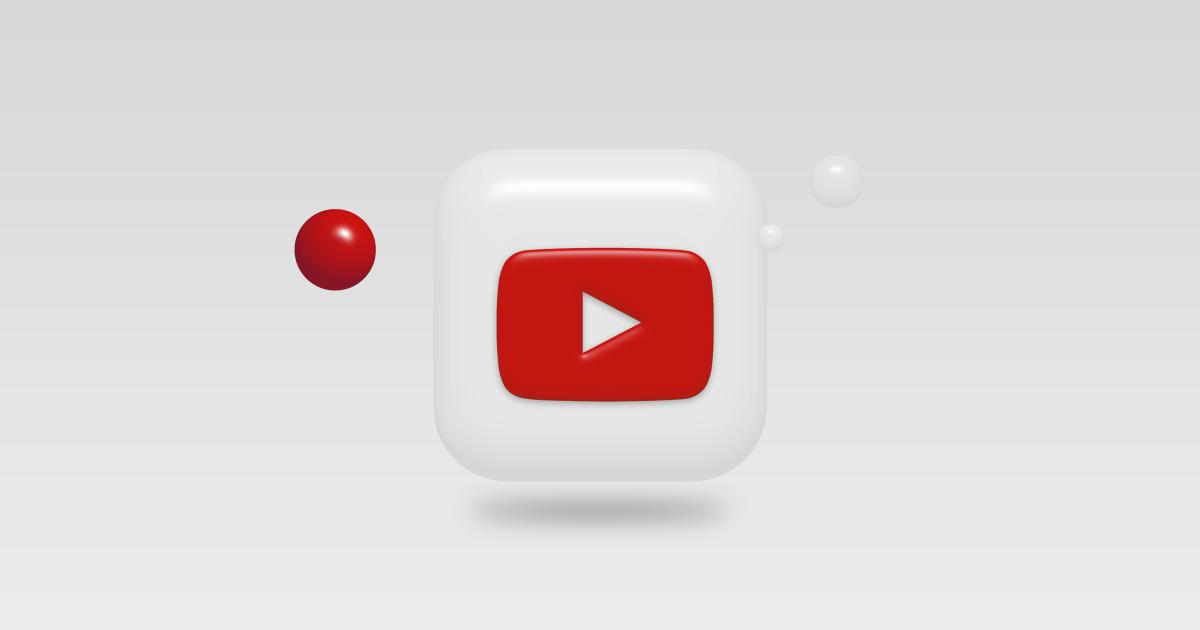
By combining server push with efficient caching and conditional requests, you can further enhance the performance and responsiveness of your web application.
Monitor and Measure the Impact
Continuously monitoring the performance and impact of your server push implementation is essential for optimization. Gather metrics such as page load times, resource delivery latency, and user engagement to assess the effectiveness of your server push strategies.
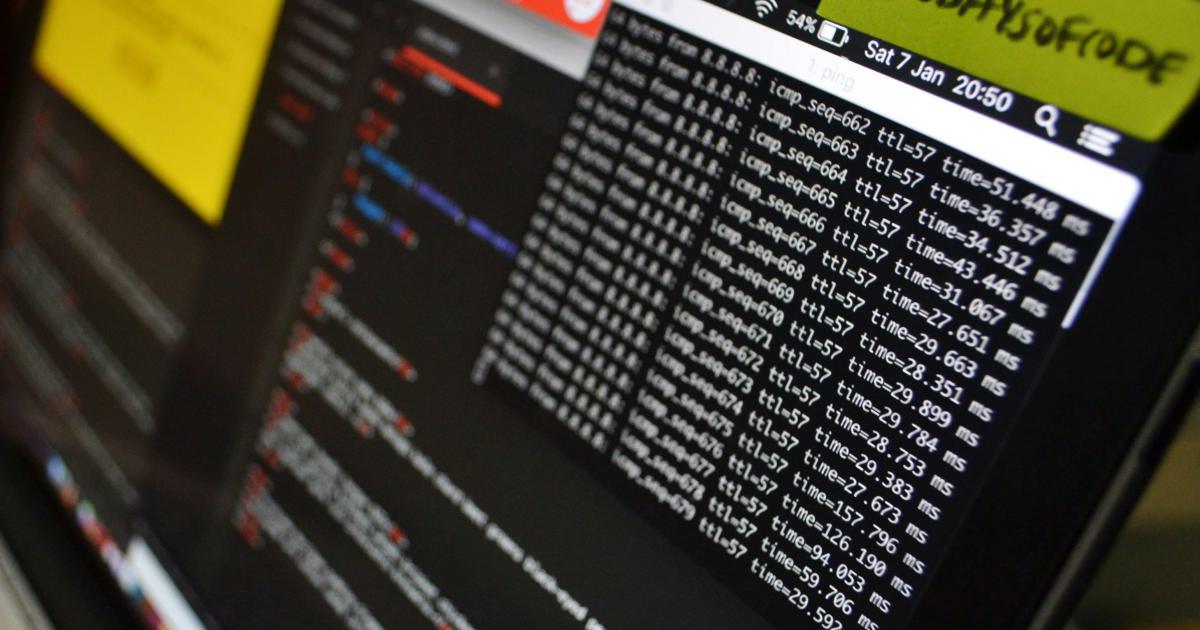
Tools like web performance monitoring services, browser developer tools, and server-side logging can provide valuable insights into the real-world impact of your server push implementation. Use these metrics to identify areas for improvement and refine your strategies accordingly.
Consider Potential Drawbacks
While server push can be a powerful technique, it's important to be aware of potential drawbacks and limitations:
Increased Server Load: Pushing resources proactively can increase the processing and bandwidth requirements on the server, potentially leading to scalability challenges.
Potential Inefficiencies: If the client already has the pushed resources cached, the server push process can result in unnecessary network traffic and resource consumption.
Browser Support Considerations: Not all browsers may support or properly handle server push. Ensure that your implementation is compatible with the target audience's browser capabilities.
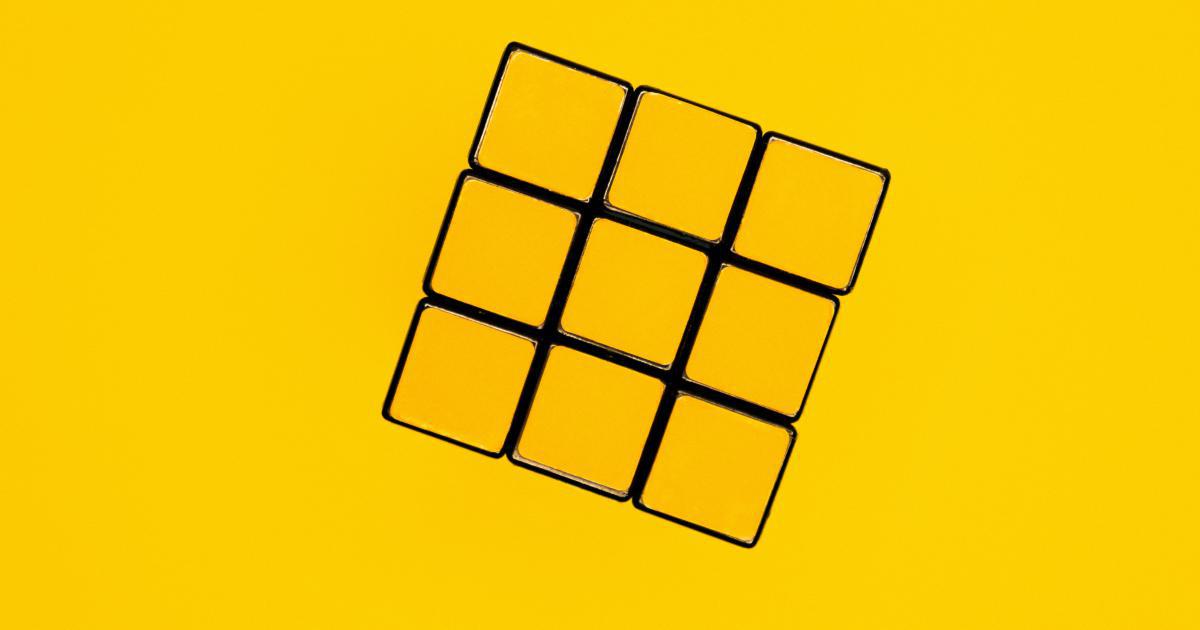
Carefully evaluate the trade-offs and potential drawbacks of server push, and integrate appropriate fallbacks or alternative strategies to ensure a consistent user experience across all supported browsers.
Practical Applications of HTTP/2 Server Push
Improving Page Load Times
One of the primary use cases for HTTP/2 server push is to improve the perceived performance of web pages. By proactively delivering critical resources, such as CSS, JavaScript, and images, the server can reduce the number of round-trips required to fully load a page, leading to faster load times and a more responsive user experience.
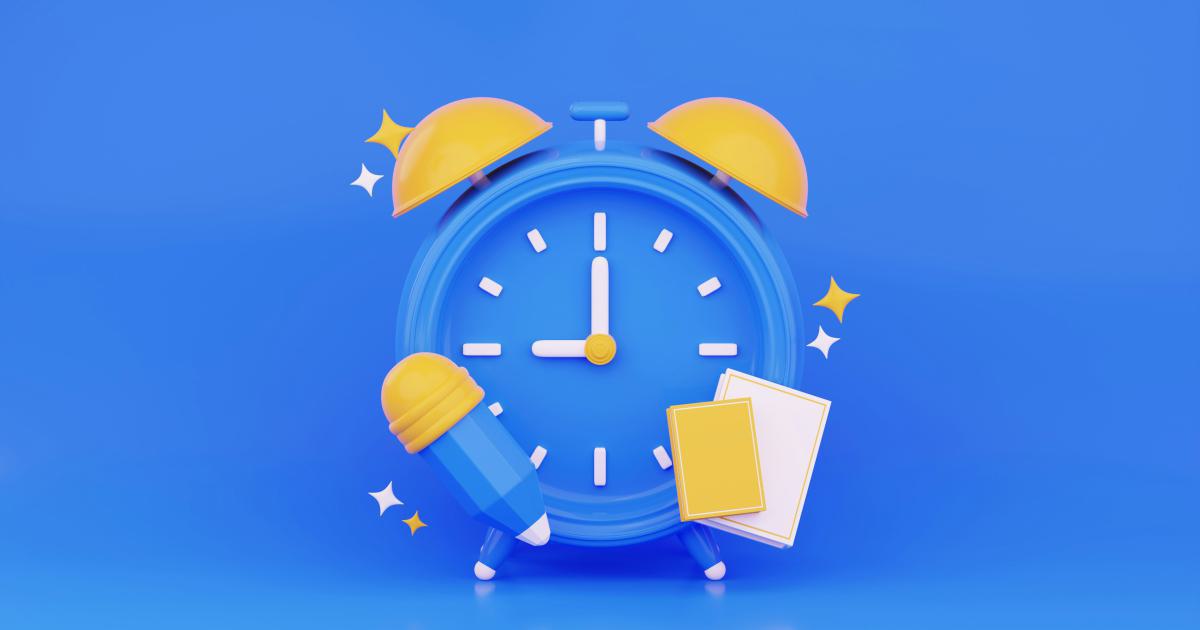
This is particularly beneficial for resource-heavy pages, where the client would otherwise need to make multiple requests to fetch all the necessary assets. Server push can be especially impactful for mobile users, who often experience slower network conditions and higher latency.
Enhancing Single Page Applications (SPAs)
In the context of Single Page Applications (SPAs), server push can be a valuable tool for optimizing the initial page load and subsequent navigations within the application.
For the initial page load, the server can identify and push the resources required to render the initial view, allowing the SPA to bootstrap and become interactive more quickly.
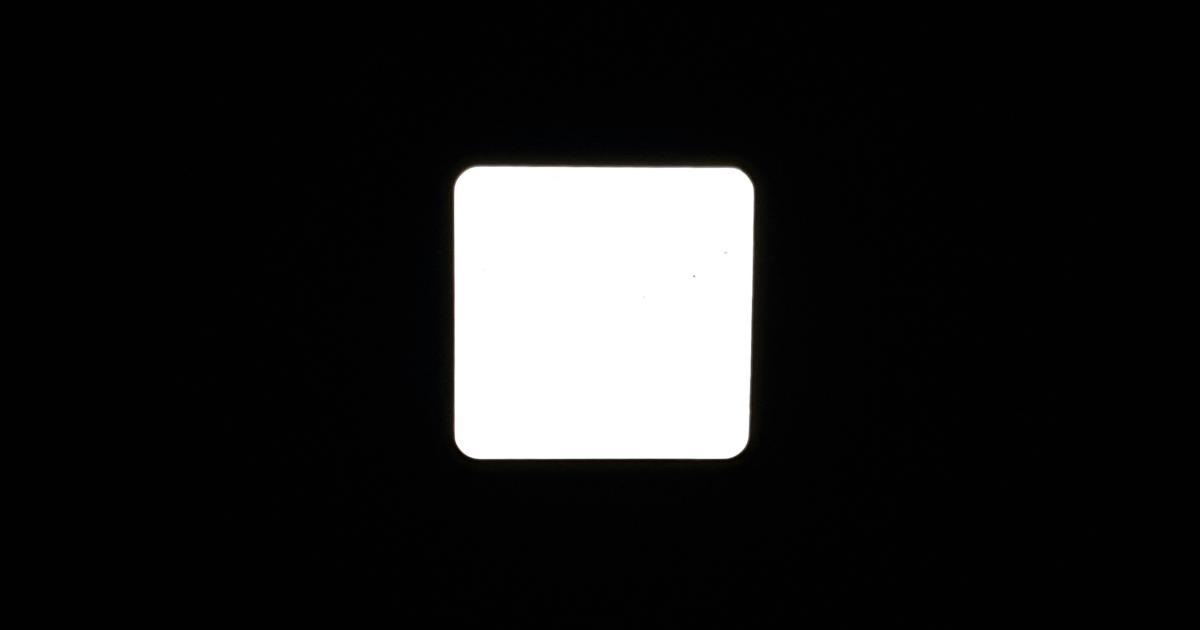
As the user navigates within the SPA, the server can also anticipate the resources needed for the next views and proactively push them, minimizing the perceived latency between page transitions.
Delivering Progressive Web Apps (PWAs)
Progressive Web Apps (PWAs) are a modern web application architecture that aims to provide an app-like experience on the web. Server push can be instrumental in enhancing the performance and responsiveness of PWAs, particularly during the initial load and subsequent updates.
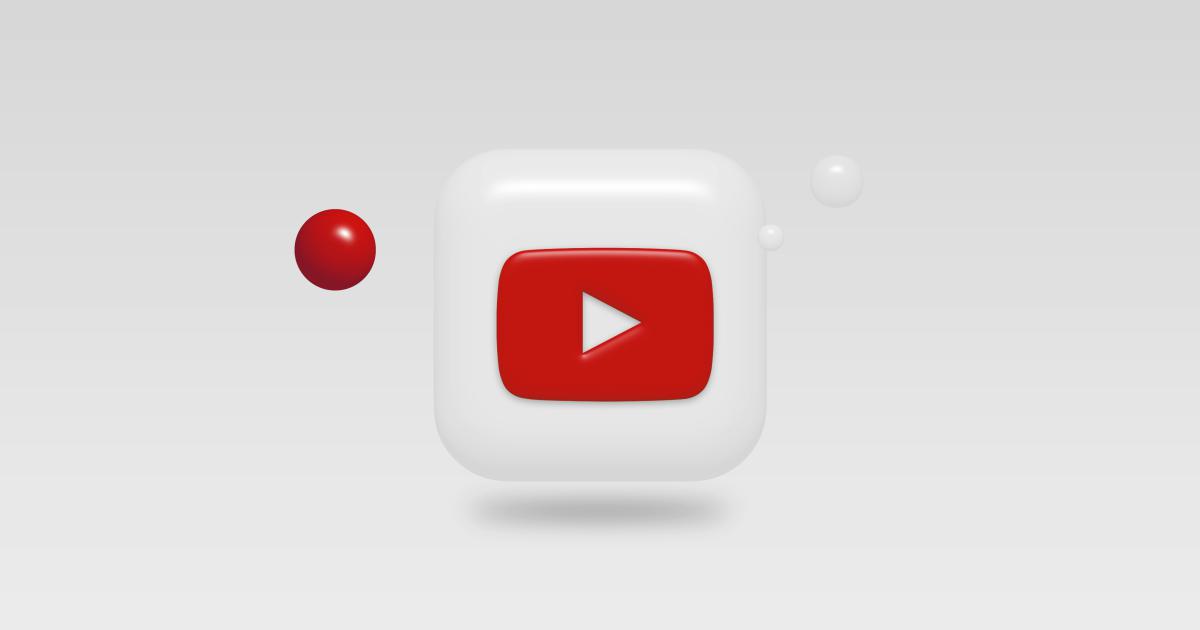
By pushing the critical resources required for the PWA's core functionality, the server can ensure a fast and seamless initial load. Furthermore, server push can be used to efficiently deliver updates and new content to the PWA, reducing the perceived latency experienced by users.
Optimizing Content Delivery Networks (CDNs)
HTTP/2 server push can also be leveraged in conjunction with Content Delivery Networks (CDNs) to further improve the performance of web applications.
When a client requests a resource from a CDN, the CDN server can analyze the request and proactively push any related assets, such as images, fonts, or other static content. This can significantly reduce the number of round-trips required to fully load the requested resource, leading to faster delivery times.
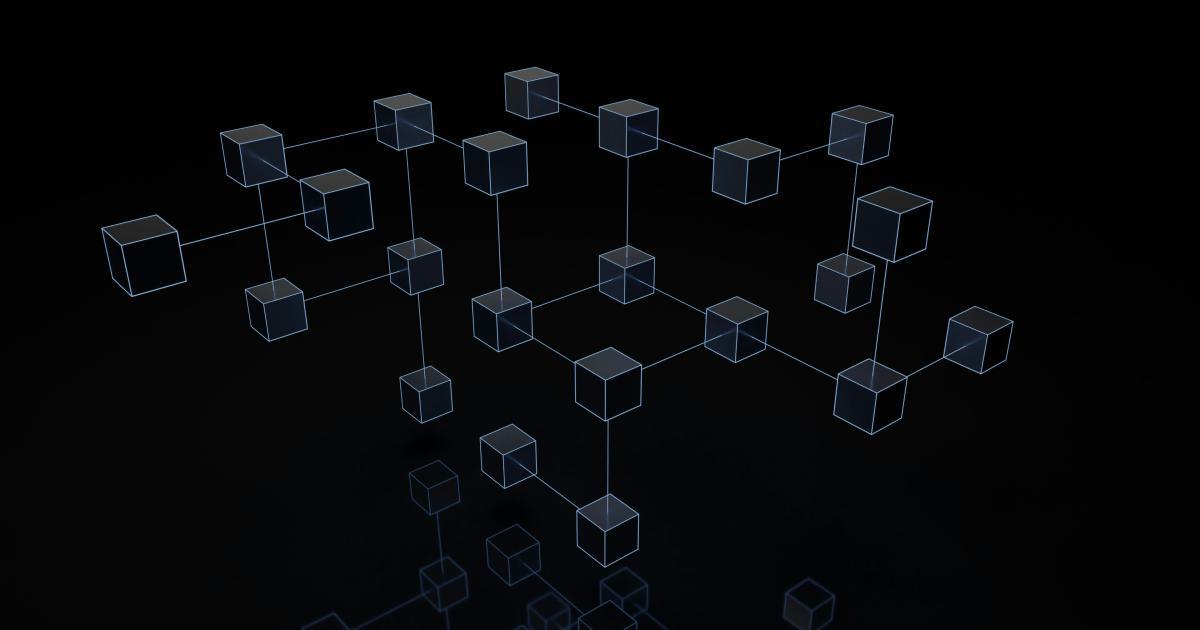
By integrating server push into your CDN strategy, you can create a more efficient and responsive content delivery ecosystem, providing users with a consistently fast and smooth web experience.
Challenges and Considerations
Browser Support and Adoption
While HTTP/2 server push is a powerful feature, its adoption and support across different browsers can vary. Some older browsers may not support the HTTP/2 protocol or may not properly handle the server push mechanism, leading to potential compatibility issues.
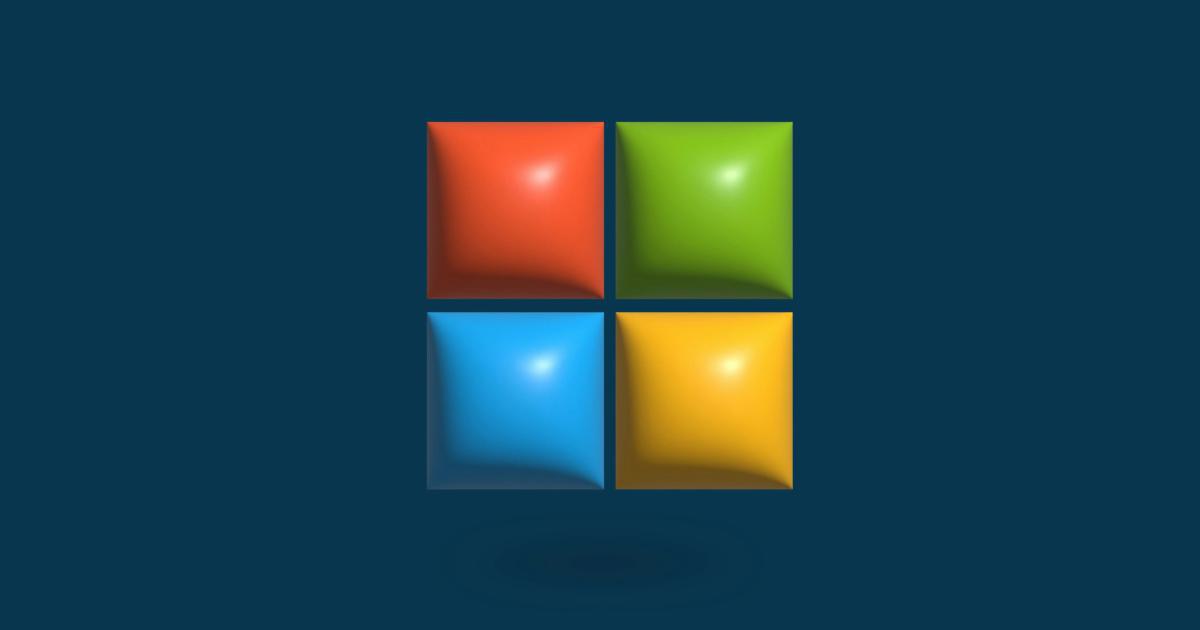
It's essential to monitor the browser landscape, stay up-to-date with the latest developments, and implement appropriate fallbacks or alternative strategies to ensure a consistent user experience across all supported browsers.
Managing Resource Dependencies
Effectively managing resource dependencies is crucial when implementing server push. If the server pushes resources that are not immediately needed by the client, it can lead to increased network traffic and resource consumption, potentially negating the benefits of server push.
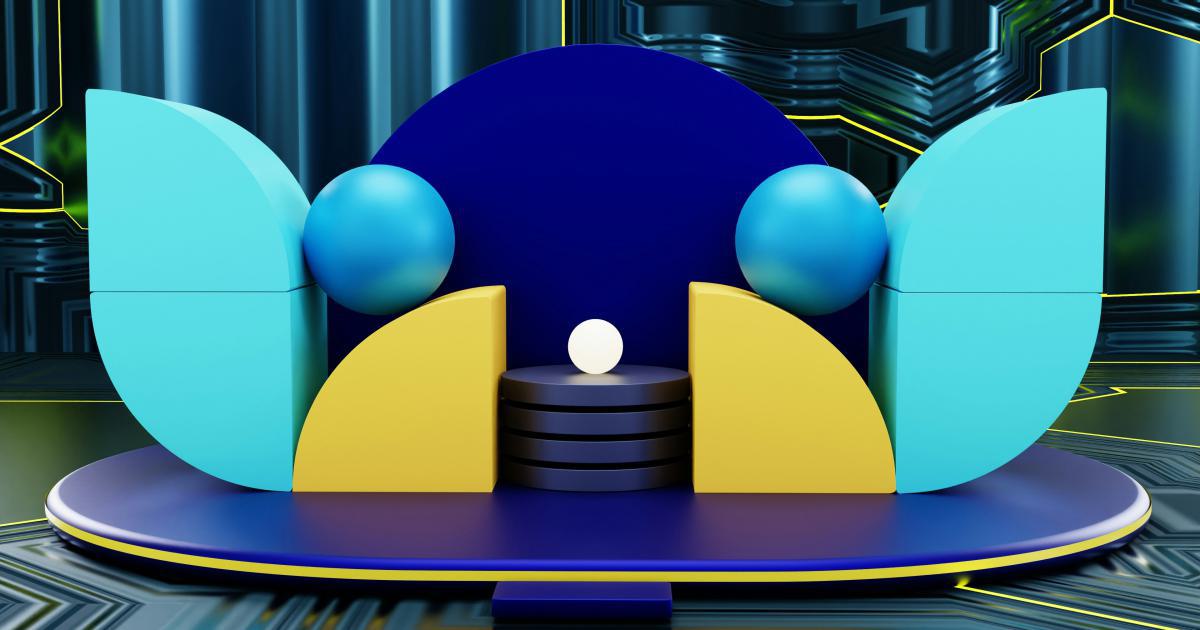
Carefully analyze the relationships between different resources and ensure that the pushed assets align with the client's requirements. Utilize techniques like code splitting, lazy loading, and conditional push strategies to optimize the resource delivery process.
Measuring and Monitoring Performance
Accurately measuring the performance impact of server push can be challenging, as it involves analyzing various metrics and understanding the complex interactions between the client and server.
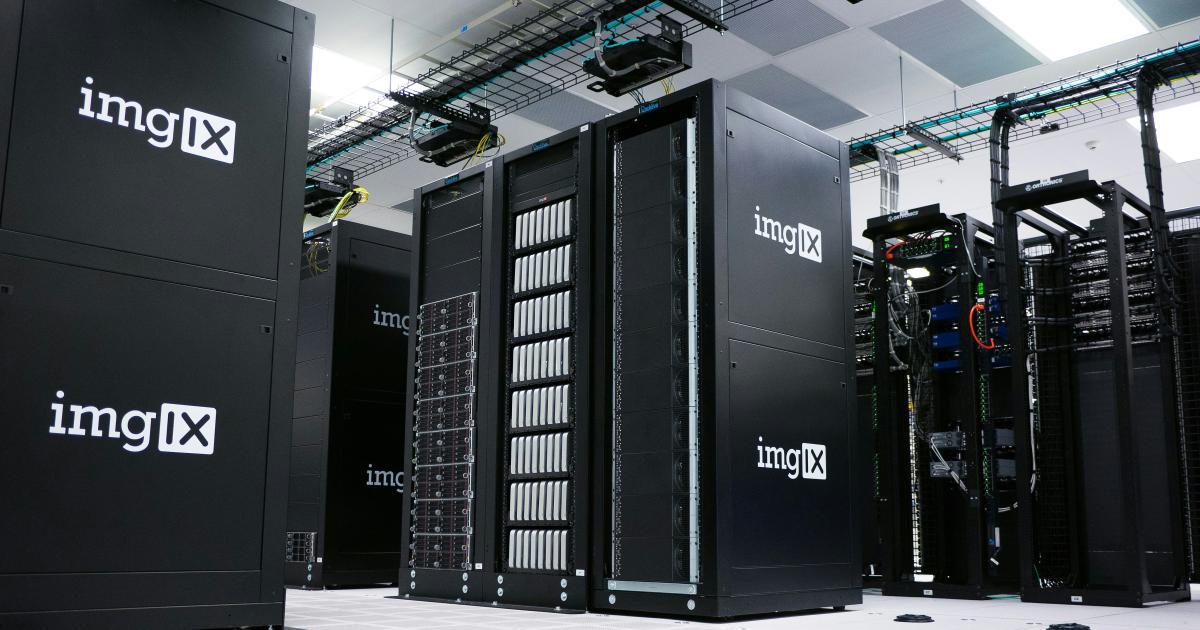
Employ a combination of client-side and server-side monitoring tools to gather comprehensive data on page load times, resource delivery latency, and user engagement. Continuously analyze and refine your server push strategies based on the insights gained from these performance metrics.
Balancing Server Load and Efficiency
While server push can improve the user experience, it can also increase the processing and bandwidth requirements on the server. Striking the right balance between server load and efficiency is crucial to ensure the scalability and sustainability of your web application.
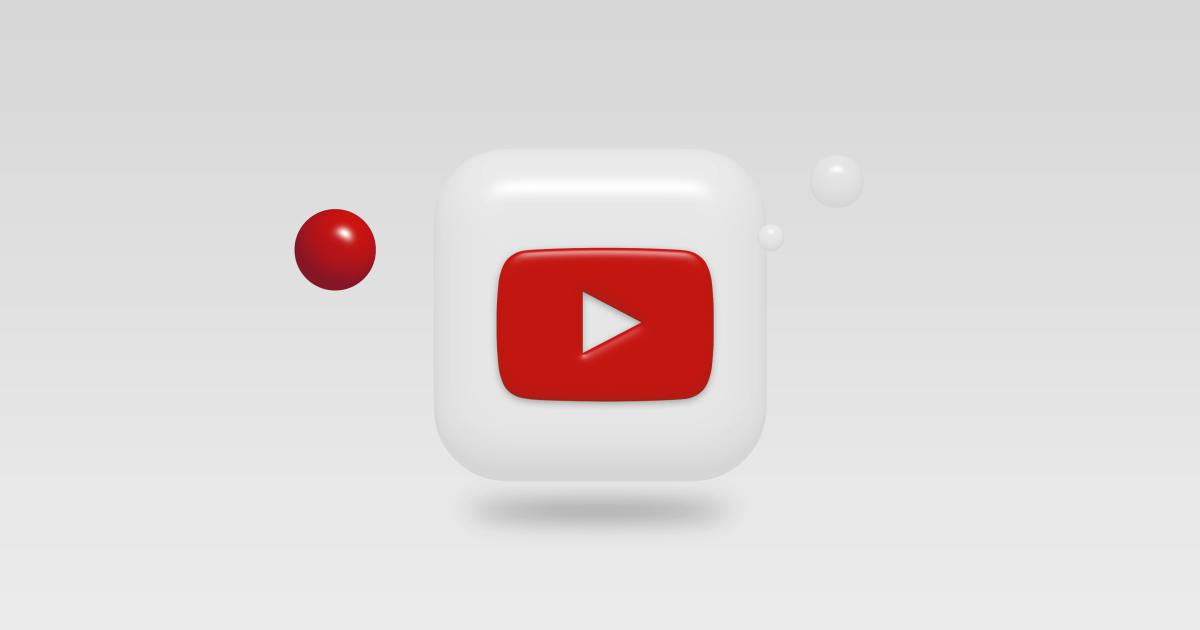
Monitor server resource utilization, analyze the trade-offs between pushed resources and performance gains, and implement caching strategies and load-balancing mechanisms to maintain a healthy and efficient server infrastructure.
Conclusion: Mastering HTTP/2 Server Push
In the ever-evolving landscape of web development, HTTP/2 server push has emerged as a powerful technique for improving the performance and responsiveness of web applications. By leveraging this capability, web developers and architects can deliver a more efficient and seamless user experience, ultimately enhancing overall customer satisfaction and engagement.
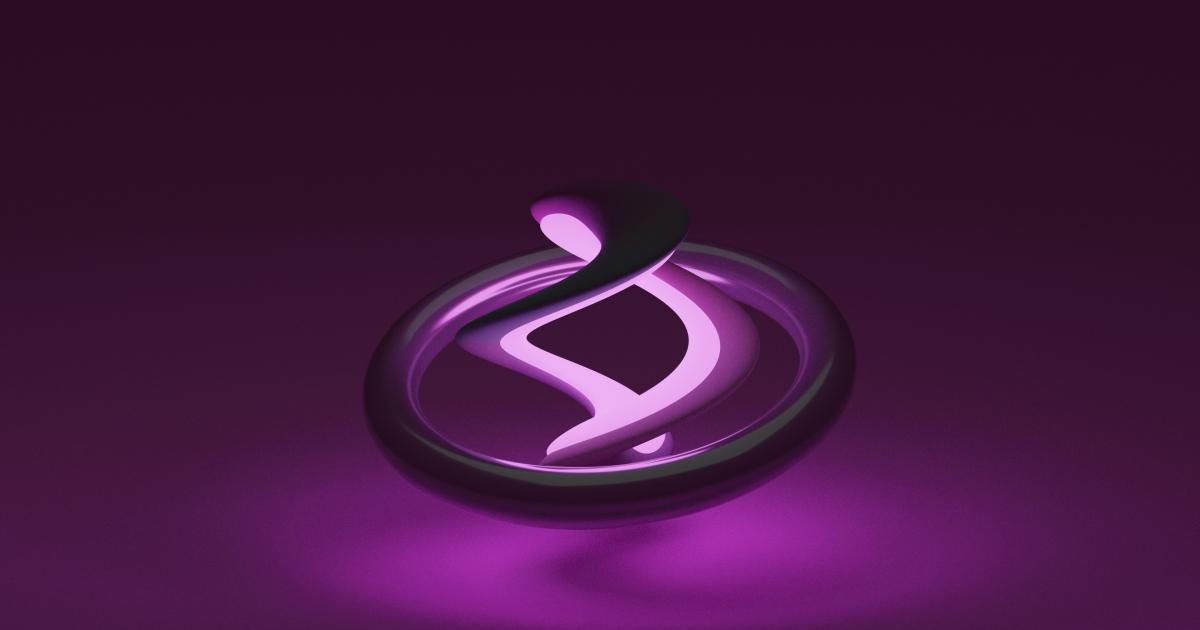
As you continue to explore and implement HTTP/2 server push, remember to stay vigilant, continuously monitor your performance metrics, and adapt your strategies based on the unique needs and challenges of your web application. By mastering this technology, you'll be able to unlock new levels of optimization and deliver web experiences that truly stand out in the digital world.
Further Reading
- HTTP/2 Specification
- HTTP/2 Server Push: The Complete Guide
- Implementing HTTP/2 Server Push in Node.js
- Best Practices for HTTP/2 Server Push
- Measuring the Impact of HTTP/2 Server Push